前言
有时候,我们需要对一个目录下的所有图片都进行重命名,如果你选择手动方式进行,在图片少的情况下还可以进行,但是如果一个目录下有几百张图片时,你就会感到无比痛苦了。这时候就会想借助工具来实现了。如下就是一种很简单的实现方式。
实现
可以选择直接下载这个jar包工具,然后通过 java -jar xxx.jar
的方式运行,也可以直接看其源代码然后拷贝其rename方法运行即可。
代码已托管在gitee上,请移步查看。
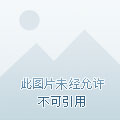
流操作之下载图片
@Test public void downloadImg() throws Exception{ String urlStr = "https://images7.alphacoders.com/118/1180526.jpg"; URL url = new URL(urlStr); HttpURLConnection connection = url.openConnection(); InputStream is = connection.getInputStream(); File file = new File("e:/temp-files/1.png"); FileOutputStream fos = new FileOutputStream(file); byte[] buffer = new byte[1024]; int len = 0; while((len = is.read(buffer)) != -1){ fos.write(buffer, 0, len); } fos.close(); is.close(); System.out.println("download completed"); }
|
流操作之复制图片
@Test public void copyImg() throws Exception{ String imgPath = "e:/temp-files/1.png"; FileInputStream is = new FileInputStream(imgPath); File file = new File("e:/temp-files/1-bak.png"); FileOutputStream fos = new FileOutputStream(file); byte[] buffer = new byte[1024]; int len = 0; while((len = is.read(buffer)) != -1){ fos.write(buffer, 0, len); } fos.close(); is.close(); System.out.println("copy img completed"); }
|
流操作之复制txt
@Test public void copyTxt() throws Exception{ String filePath = "e:/temp-files/1.txt"; FileInputStream fis = new FileInputStream(filePath); File file = new File("e:/temp-files/1-bak.txt"); FileOutputStream fos = new FileOutputStream(file); byte[] buffer = new byte[1024]; int len = 0; while ((len = fis.read(buffer)) != -1){ fos.write(buffer, 0, len); } fos.close(); fis.close(); System.out.println("txt copy completed"); }
|
通过工具图片API获取图片
import com.alibaba.fastjson.JSON; import com.alibaba.fastjson.JSONObject; import org.apache.http.client.methods.CloseableHttpResponse; import org.apache.http.client.methods.HttpGet; import org.apache.http.impl.client.CloseableHttpClient; import org.apache.http.impl.client.HttpClientBuilder; import org.apache.http.util.EntityUtils;
public static String getImg() { String str = ""; try { String urlStr = "https://www.dmoe.cc/random.php?return=json"; CloseableHttpClient httpClient = HttpClientBuilder.create().build(); HttpGet httpGet = new HttpGet(urlStr); CloseableHttpResponse response = httpClient.execute(httpGet); String responseString = EntityUtils.toString(response.getEntity(), "UTF-8"); JSONObject responseJson = JSON.parseObject(responseString); System.out.println(responseJson); str = responseJson.get("imgurl").toString(); } catch (Exception e) { e.printStackTrace(); } return str; }
|